When I decided to join the tech world and I started job hunting I saw SQL in almost every single requirements section. I tried to learn it online but it just seemed so difficult and with all the other programs that one was expected to know, I was overwhelmed. I'm very happy to say that I now understand it way better and it's so not as hard as people make it out to be sometimes.
SQL stands for Structured Query Language. Just as groups of people have languages they can speak to communicate with each other, computers need a way to communicate with each other. SQL lets you access and manipulate databases. What this means is that you can:
- Ask questions of databases and have the answering data returned to you
- Add, delete and update rows of data in your database
- Create new tables in your database or even create a new database
- Create procedures and views and set user permissions
Now, the BASICS!!
The order of operations is incredibly important with SQL:
- Select - This is where you say what you want. You could want every row (use the wildcard *) or you could want specific columns only
- From - This is where you indicate the table that contains the information from above.
The table below is called Customers.
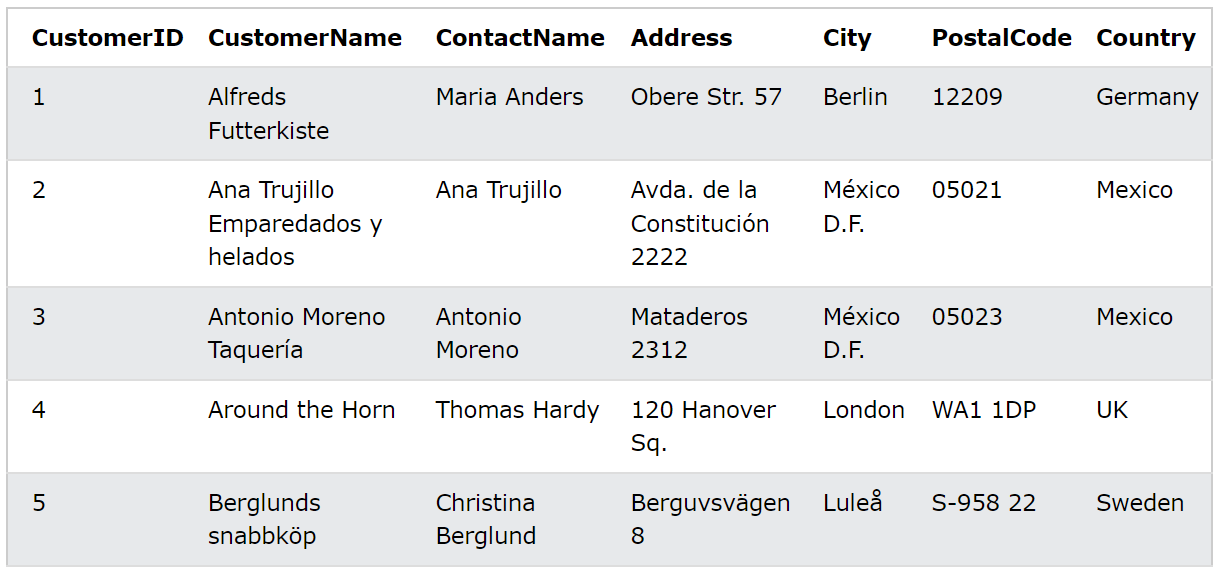
If my SQL statement is:
SELECT CustomerName,City
FROM Customers;
This is the result I would get:
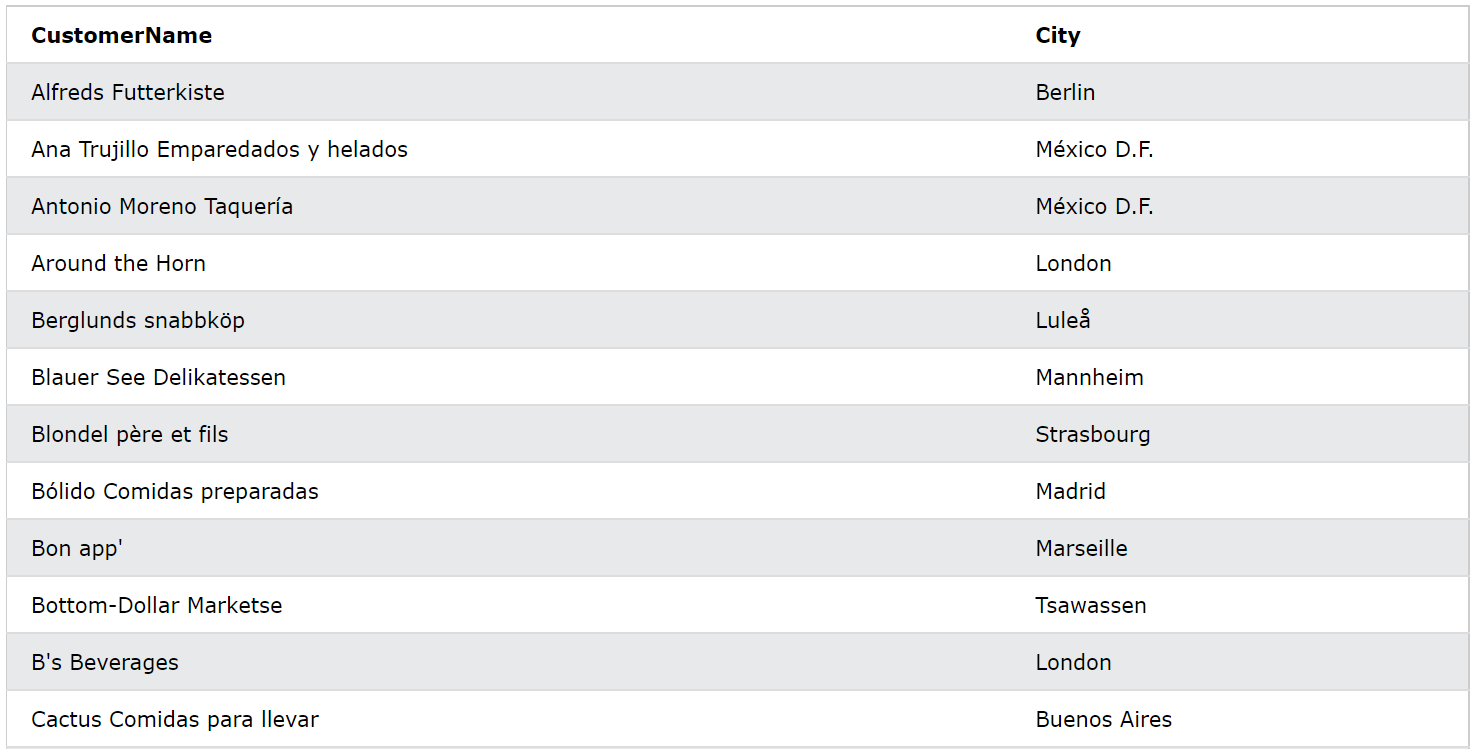
Only the two column names I identified were returned. Using the * wildcard would have given me the entire table.
3. Where - This filters the records that you get back.
If my SQL statement is:
SELECT *
FROM Customers
WHERE Country='Mexico';
This means that I want all columns from the Customers table but I only want the rows that have Mexico in the country column. So i'm filtering for Mexico.
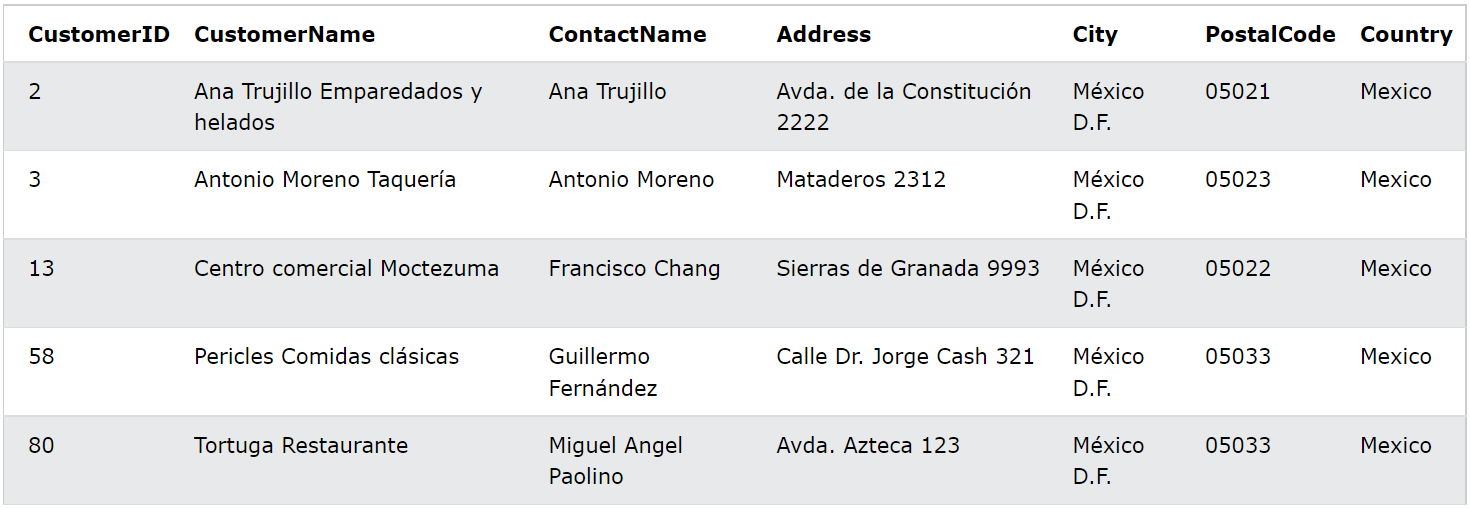
You can also combine the WHERE clause with AND, OR and NOT operators. I can filter for Country='Mexico' AND City='Berlin'

I can filter for City='Berlin' OR City='München'

I can filter for NOT Country='Germany' (this means I want the records where the country isn't Germany)
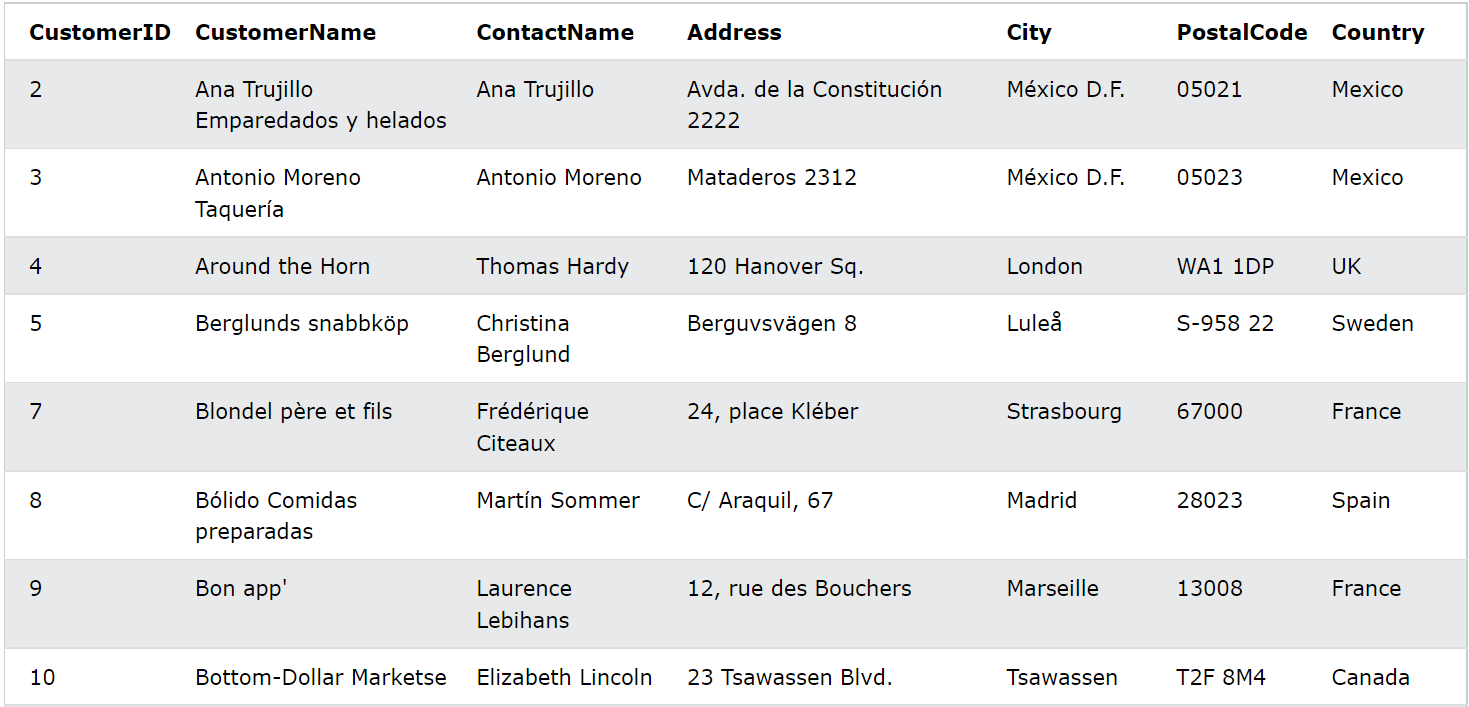
4. Group By - This let's you summarise the information and you get a count of how many records fit in each group.
My SQL statement:
SELECT COUNT(CustomerID), Country
FROM Customers
GROUP BY Country;
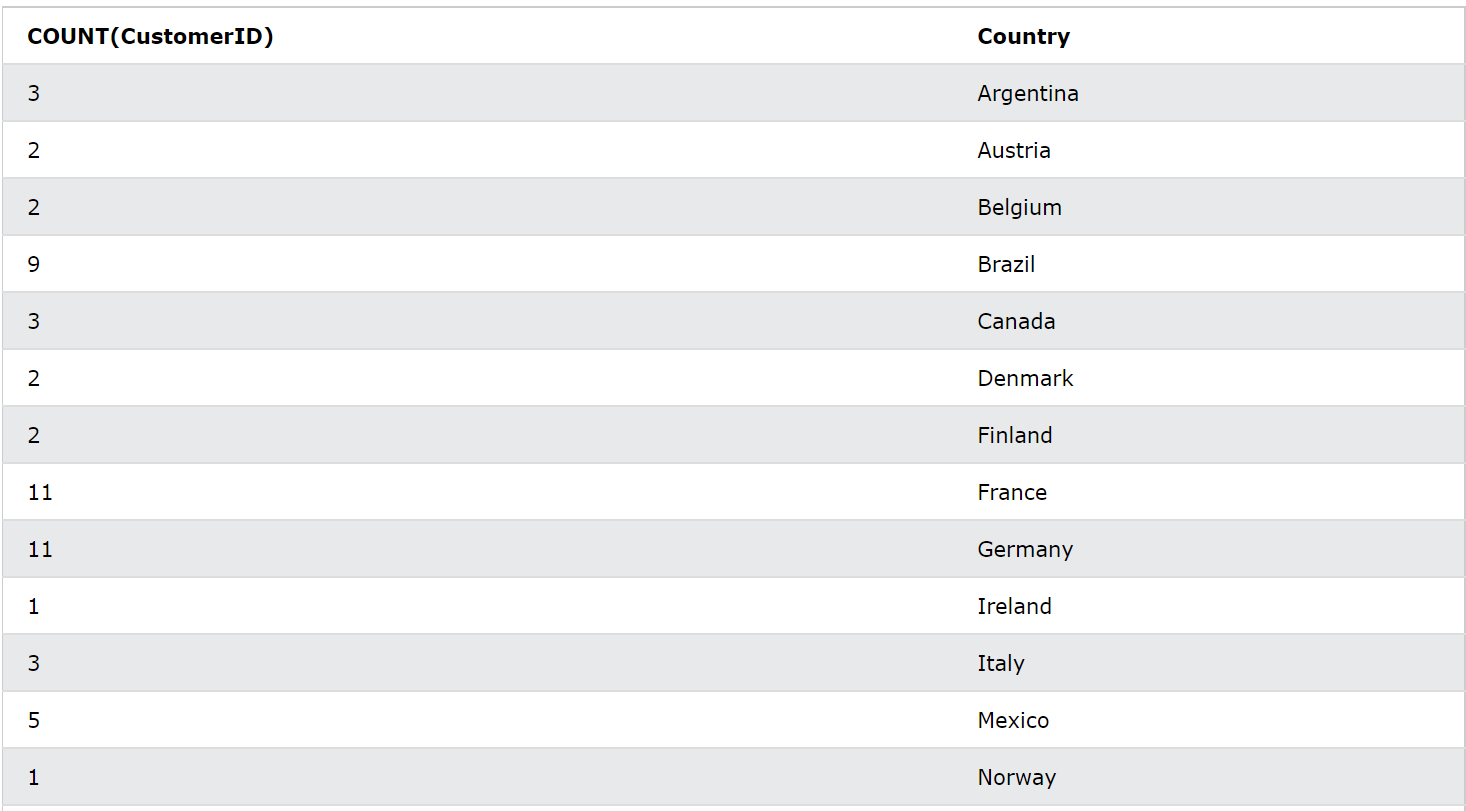
5. Having - This is used when there are aggregate functions being used and we can't use the WHERE clause. WHERE filters the data on a row by row basis and after aggregating, this can't happen anymore.
My SQL statement (COUNT is the aggregator here):
SELECT COUNT(CustomerID), Country
FROM Customers
GROUP BY Country
HAVING COUNT(CustomerID) > 5;
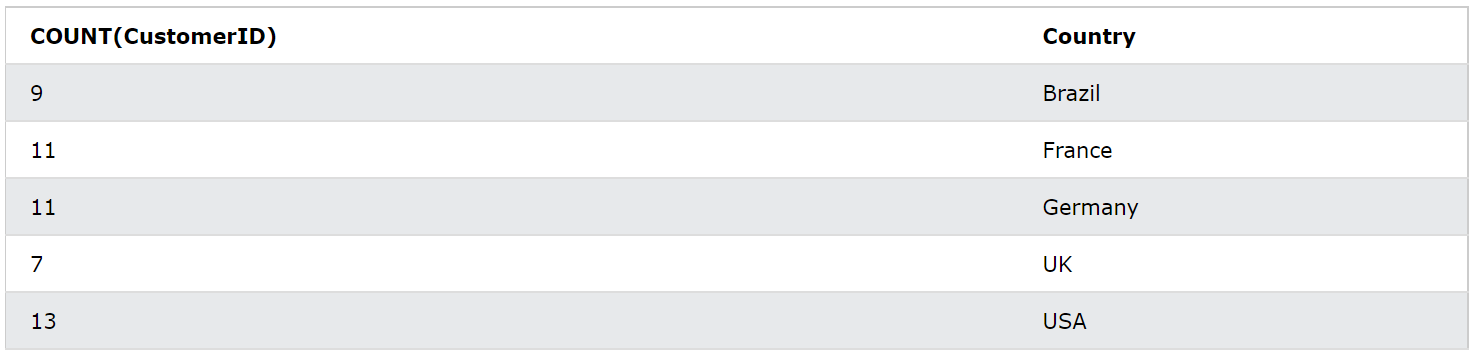
6. Order By - This is used to sort the data we get back from our query in either ascending and descending order
My SQL statement:
SELECT * FROM Customers
ORDER BY Country;
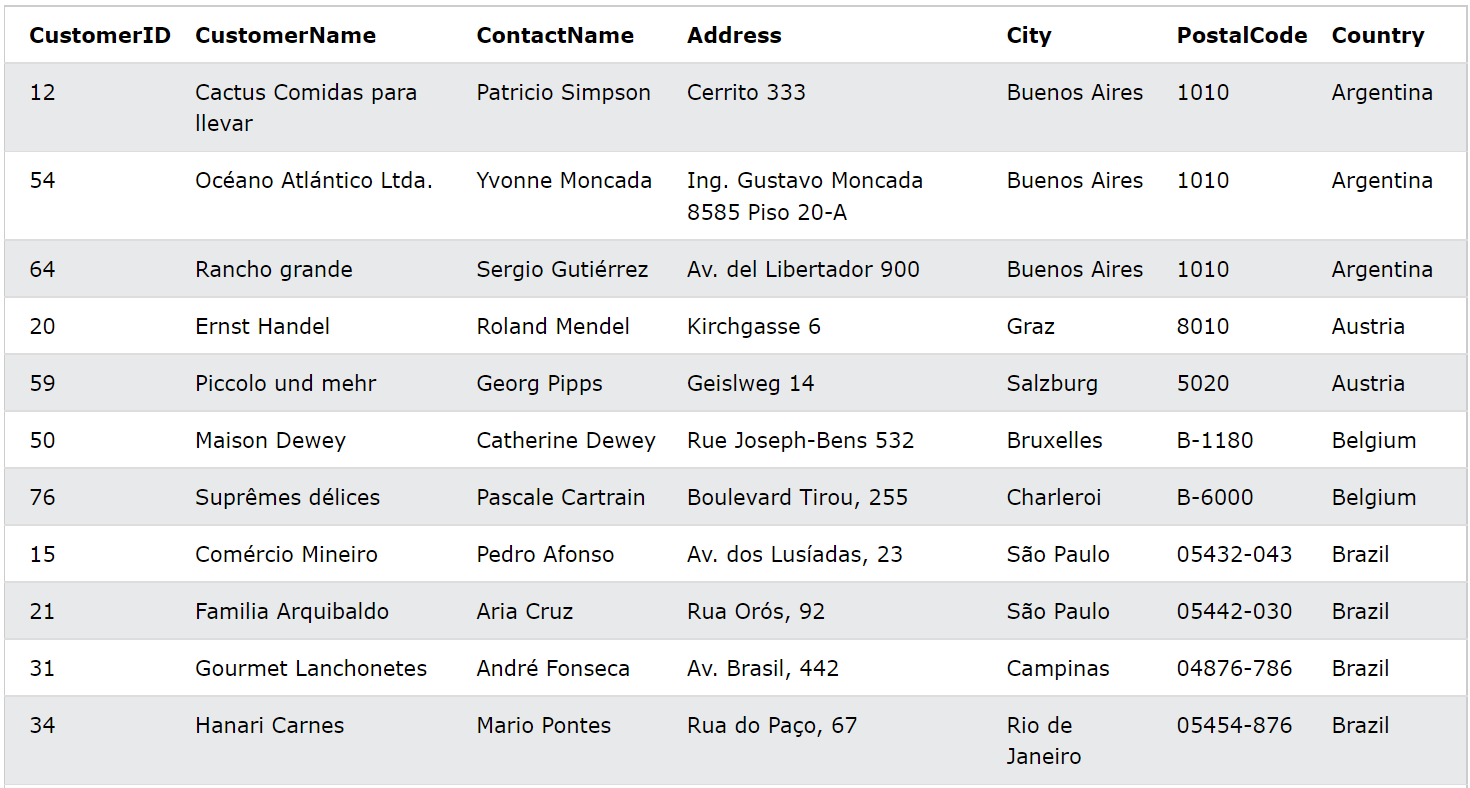
There are a tonnes of other things you can do with SQL but these are the basics and you can get a lot of practice out of them.
Watch this space for more SQL content 😊
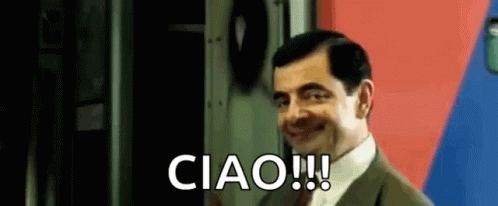